PHP is a general-purpose scripting language that is especially suited for web development. It is fast, efficient, and easy to learn. PHP is also open source, which means that it is free to use and distribute.
This guide will teach you everything you need to know to get started with PHP web development. You will learn about the basics of PHP, such as variables, operators, and control structures. You will also learn about more advanced topics, such as object-oriented programming and database development.
By the end of this guide, you will be able to create your own PHP web applications.
PHP Web Development Popularity
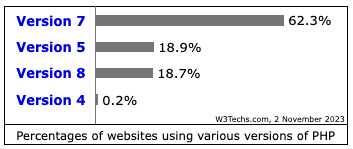
Source: W3Techs
- PHP is used by 76.8% of all the websites whose server-side programming language we know.
- Version 7 is used by 62.3% of all the websites who use PHP.
- The PHP programming language powers 45% of all internet websites, as WordPress uses it.
What is PHP?
PHP is a server-side scripting language that is used to create dynamic web pages. This means that PHP code is executed on the web server, and the results are then sent to the user’s browser. This is in contrast to client-side scripting languages, such as JavaScript, which are executed on the user’s browser.
PHP is embedded into HTML, which means that PHP code can be placed anywhere within an HTML document. When a user requests an HTML page that contains PHP code, the web server executes the PHP code and then sends the results, along with the rest of the HTML page, to the user’s browser.
PHP is a very powerful tool for creating web applications because it allows developers to create dynamic web pages that can interact with databases and other resources on the web server. For example, PHP can be used to create web pages that allow users to log in, submit forms, and view personalized content.
Why use PHP?
- PHP is free and open source, which means that anyone can use it and contribute to its development. This has led to a large and active community of PHP developers who create and share libraries, frameworks, and other resources. This makes it easy for new developers to get started with PHP and to find help when they need it.
- PHP is also easy to learn and use. The syntax is relatively simple and straightforward, and there are many resources available to help new developers learn the language. PHP is also very forgiving of errors, which makes it a good choice for beginners.
- PHP is fast and efficient. It is designed to be used on web servers, and it is very good at handling large volumes of traffic. PHP is also relatively resource-efficient, which means that it can be used on smaller servers.
- PHP is secure. PHP has a number of built-in security features that help to protect web applications from attacks. For example, PHP provides support for input validation, output filtering, and session management.
- PHP is scalable. PHP applications can be scaled to handle large numbers of users and transactions. This is because PHP is designed to be efficient and to use resources wisely.
- PHP is extensible. PHP can be extended with frameworks, libraries, and modules. This makes it easy to add new features and functionality to PHP applications.
- PHP is compatible with a wide range of web servers and databases. This makes it a good choice for developers who need to deploy their applications on a variety of platforms.
Choosing a PHP framework
Factors to consider when choosing a PHP framework:
Features:
Consider the features that are important to you in a PHP framework. Some frameworks offer a wide range of features, such as built-in authentication, session management, and database access. Other frameworks are more lightweight and focus on providing a core set of features that can be extended with libraries and modules.
Performance:
PHP frameworks can vary in terms of performance. Some frameworks are more optimized for speed, while others are more optimized for scalability. Consider the expected load on your web application when choosing a framework.
Community support:
A large and active community is important for any PHP framework. A large community means that there are more resources available, such as documentation, tutorials, and support forums.
Maturity:
Choose a framework that is mature and well-established. This means that the framework has been around for a while and has a proven track record.
Recommendations for different types of web applications:
Small and simple web applications:
For small and simple web applications, a lightweight framework such as CodeIgniter or Slim may be a good choice. These frameworks offer a core set of features and are easy to learn.
Medium-sized web applications:
For medium-sized web applications, a full-stack framework such as Laravel or Symfony may be a good choice. These frameworks offer a wide range of features and are well-suited for developing complex web applications.
Large and enterprise-scale web applications:
For large and enterprise-scale web applications, a framework such as Symfony or Zend Framework may be a good choice. These frameworks are highly scalable and offer a wide range of features for developing robust web applications.
Examples of popular PHP frameworks:
Laravel:
Laravel is a popular PHP framework that is known for its elegance, simplicity, and ease of use. Laravel offers a wide range of features, including built-in authentication, session management, and database access. Laravel is a good choice for developing a wide variety of web applications, from small and simple to large and complex.Symfony:
Symfony is a popular PHP framework that is known for its flexibility and scalability. Symfony offers a wide range of features, including a component-based architecture, built-in security features, and a powerful template engine. Symfony is a good choice for developing complex and enterprise-scale web applications.CodeIgniter:
CodeIgniter is a popular PHP framework that is known for its simplicity and ease of use. CodeIgniter offers a core set of features, such as routing, database access, and form validation. CodeIgniter is a good choice for developing small and simple web applications.Slim:
Slim is a popular PHP framework that is known for its speed and lightweight design. Slim is a good choice for developing RESTful APIs and microservices.Zend Framework:
Zend Framework is a popular PHP framework that is known for its enterprise-grade features and robust security features. Zend Framework is a good choice for developing large and complex web applications.
Getting started with PHP:
Choose a web server and PHP interpreter.
There are many different web servers and PHP interpreters available. Some popular choices include:
- Web servers: Apache, Nginx, and IIS
- PHP interpreters: PHP 7.4, PHP 8.0, and PHP 8.1
Once you have chosen a web server and PHP interpreter, you will need to install them on your computer. There are many different ways to do this, so you will need to consult the documentation for your chosen web server and PHP interpreter.
Configure the web server.
Once the web server and PHP interpreter are installed, you will need to configure the web server to recognize PHP files. This process varies depending on the web server that you are using, so you will need to consult the documentation for your chosen web server.
For example, to configure Apache to recognize PHP files, you will need to add the following lines to the .htaccess file in the root directory of your web server:
AddHandler application/x-httpd-php .php
Create a PHP script.
A PHP script is a text file that contains PHP code. The file must have the .php extension. To create a PHP script, open a text editor and create a new file. Save the file with the .php extension.
For example, to create a simple PHP script that prints the message “Hello, world!” to the browser, you would create a new file called hello_world.php and save it in the root directory of your web server. The contents of the file would be as follows:
<?php
echo "Hello, world!";
?>
Run the PHP script.
To run the PHP script, simply open the file in a web browser. The web browser will execute the PHP code and then display the results.
For example, to run the hello_world.php script, you would open the file in a web browser by navigating to the following URL:
http://localhost/hello_world.php
If everything is configured correctly, the browser will display the message “Hello, world!”
Write some PHP code.
In the PHP script, you can write any valid PHP code. The simplest PHP code is the echo statement, which prints the specified text to the browser. However, PHP can be used to do much more than just print text to the browser.
For example, PHP can be used to:
- Connect to databases
- Process forms
- Generate dynamic web pages
- Send emails
- And much more
Example of a simple PHP web application:
<?php
// Connect to the database
$db = new PDO('mysql:host=localhost;dbname=my_database', 'root', '');
// Get the list of all users
$users = $db->query('SELECT * FROM users');
// Display the list of users
foreach ($users as $user) {
echo $user['name'] . '<br>';
}
// Close the database connection
$db = null;
?>
This code will connect to a MySQL database and retrieve a list of all users. It will then display the list of users on the web page.
To deploy this web application, you would need to upload the code to a web server and create a database on the web server. You would then need to configure the web server to recognize PHP files.
Once the web application is deployed, you would be able to access it by visiting the following URL in a web browser:
http://https://www.instructables.com/Set-up-your-very-own-Web-server//index.php
This would display the list of users in the database.
Summary Highlights
PHP is a powerful and versatile language that is perfect for web development. It is easy to learn, efficient, and free to use. It is also supported by a large community of developers.
If you are looking to develop a web application, PHP is a great choice. It can be used to develop a wide variety of web applications, from small and simple to large and complex.
If you need help developing your PHP web application, GeekyAnts can help. GeekyAnts has a team of experienced developers who can help you with all aspects of web development, from planning and design to implementation and deployment.
Contact GeekyAnts today to learn more about their web development services.